In the world of cloud computing, managing and securing application credentials can be a challenge. Hardcoding credentials or managing secrets manually increases the risk of breaches and adds operational overhead. Managed Identities in Azure provide a seamless and secure way for your applications to access Azure resources without explicit credentials. This article dives deep into how Managed Identities work, their benefits, and how to implement them with real-world examples.
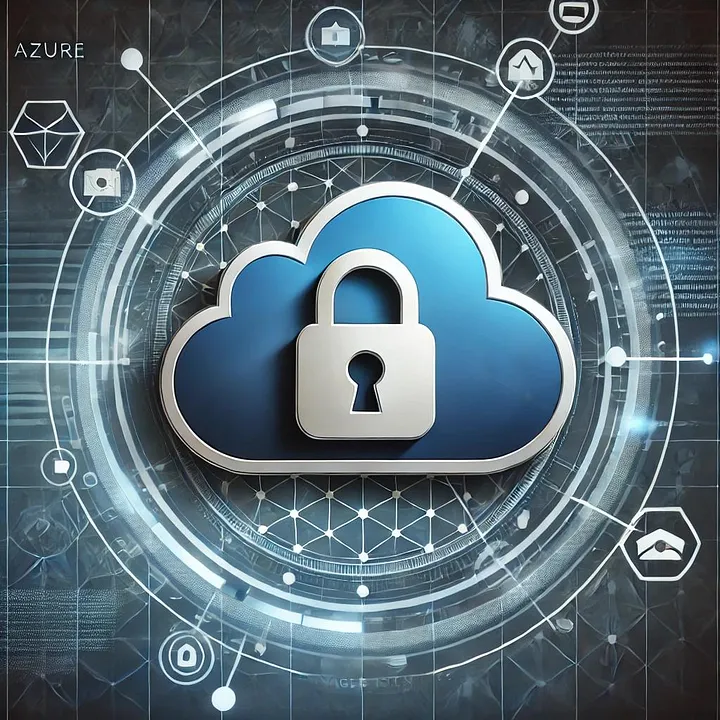
What are Managed Identities?
Managed Identities are a feature of Azure Entra ID (formerly Azure Active Directory) that automatically manages the identity of your Azure resources. When you enable a Managed Identity for a resource, Azure creates and rotates the credentials, ensuring secure communication between your application and other Azure services.
Types of Managed Identities:
- System-Assigned Managed Identity: Tied to a specific Azure resource. If the resource is deleted, the identity is also removed.
- User-Assigned Managed Identity: Created as a standalone Azure resource and can be assigned to multiple Azure resources.
Benefits of Managed Identities
- Eliminate Credential Management: There is no need to store credentials in your code or manage secret rotation.
- Enhanced Security: Reduces the risk of credential leaks by providing a secure, managed identity for Azure resources.
- Seamless Integration: Works natively with Azure services like Azure Key Vault, Azure Storage, and Azure SQL Database.
- Simplified Role Assignments: Easily assign roles to identities for fine-grained access control.
How Managed Identities Work
When you enable a Managed Identity for a resource:
- Azure generates an identity in Azure Entra ID.
- The resource can use this identity to authenticate to Azure services via OAuth2 tokens.
- The token is used to access resources based on the assigned permissions.
Setting Up a System-Assigned Managed Identity
Step 1: Enable System-Assigned Managed Identity
Let’s enable a system-assigned Managed Identity for an Azure Virtual Machine (VM).
Using Azure CLI:
# Enable System-Assigned Managed Identity on a VM
az vm identity assign --name MyVM --resource-group MyResourceGroup
Using Azure Portal:
- Navigate to your Virtual Machine.
- Under “Settings,” select “Identity.”
- Turn on the “System Assigned” toggle and save.
Step 2: Assign Permissions to System Assigned Managed Identity
Once the identity is enabled, you must assign it a role to access Azure resources.
Assigning Role to Access Azure Key Vault
# Assign Key Vault Reader role to the VM's Managed Identity
az role assignment create --assignee <ManagedIdentity_ClientID> \
--role "Key Vault Reader" \
--scope /subscriptions/<SubscriptionID>/resourceGroups/<ResourceGroupName>/providers/Microsoft.KeyVault/vaults/<KeyVaultName>
Setting Up User-Assigned Managed Identities
Step 1: Create a User-Assigned Managed Identity
Using Azure CLI
# Create a User-Assigned Managed Identity
az identity create --name MyUserAssignedIdentity --resource-group MyResourceGroup
Using Azure Portal
- Go to the Azure Portal.
- Navigate to Managed Identities under your resource group.
- Click Create, select User-Assigned, and configure the name and region.
Step 2: Assign the Identity to Azure Resources
Once created, assign the User-Assigned Managed Identity to one or more resources.
Assigning to a Virtual Machine (VM)
# Assign User-Assigned Managed Identity to a VM
az vm identity assign --resource-group MyResourceGroup --name MyVM --identities MyUserAssignedIdentity
Using Azure Portal
- Go to the VM’s Identity settings.
- Select User-Assigned under Assigned Identities.
- Add the created Managed Identity.
Step 3: Assign Permissions to the User-Assigned Managed Identity
To allow the Managed Identity to access a resource (e.g., Azure Key Vault):
# Assign a role to the User-Assigned Managed Identity
az role assignment create --assignee <UserAssignedIdentity_ClientID> \
--role "Key Vault Reader" \
--scope /subscriptions/<SubscriptionID>/resourceGroups/<ResourceGroupName>/providers/Microsoft.KeyVault/vaults/<KeyVaultName>
Accessing Azure Resources Using Managed Identity
Let’s write a Python application running on the Azure VM that fetches secrets from the Azure Key Vault using its Managed Identity.
Prerequisites:
- Azure CLI installed for local testing.
- Azure Identity and Azure Key Vault client libraries installed:
pip install azure-identity azure-keyvault-secrets
Python Code Example:
from azure.identity import DefaultAzureCredential
from azure.keyvault.secrets import SecretClient
# Key Vault URL
key_vault_url = "https://<YourKeyVaultName>.vault.azure.net/"
# Use DefaultAzureCredential to authenticate using the Managed Identity
credential = DefaultAzureCredential()
client = SecretClient(vault_url=key_vault_url, credential=credential)
# Retrieve a secret from the Key Vault
secret_name = "MySecret"
retrieved_secret = client.get_secret(secret_name)
print(f"Secret Value: {retrieved_secret.value}")
DefaultAzureCredential
automatically detects and uses the Managed Identity of the VM.- The
SecretClient
fetches the secret from Azure Key Vault.
Best Practices
- Use System-Assigned Managed Identity for Single Resource: Use system-assigned identities when the identity is tied to the lifecycle of a single resource.
- Leverage User-Assigned Managed Identity for Shared Resources: If multiple resources need the same identity, use user-assigned Managed Identities.
- Minimize Permissions: Follow the principle of least privilege by assigning the minimum necessary permissions.
- Monitor Usage: Use Azure Monitor and Azure Activity Logs to track token usage and access attempts.
- Secure Network Access: Combine Managed Identities with Virtual Network (VNet) and Private Endpoints for enhanced security.
Troubleshooting Common Issues
1. Token Acquisition Fails
- Ensure the Managed Identity is enabled and correctly assigned.
- Verify role assignments and permissions for the identity.
2. Access Denied Errors
- Check if the Managed Identity has the necessary permissions for the resource.
- Use Azure CLI to verify role assignments.
az role assignment list --assignee <ManagedIdentity_ClientID>
Conclusion
Managed Identities simplify secure access to Azure resources, eliminating the need for manual credential management. By following the steps and best practices outlined in this article, you can integrate Managed Identities into your applications and leverage their security and operational benefits.